Loops
It can be very tedious for someone to write out code that needs to be repeated on more than occasion. Likewise, it can be impossible to predict how many times we want some code to repeated. REPEAT, WHILE and FOR are statements that enable us to avoid these kind of situations, and without them, programming would be almost certainlly a very dull task.
The WHILE statement is the most conventional form of a loop. If you want to execute lines of code repeatedly, but you unsure if you want to execute them in the first place, this is the loop to choose. While a certain condition is true, the code is repeated.
a:=1;
while (a<=3)
begin
writeln(a);
a:=a+1;
end;
You can see by this example, the integer(a) is continuously output on screen until it is equal to or less than 3. Therefore, the numbers 1, 2 and 3 should be written on each line. You can see that this can be particularly useful when you need to ensure some code is repeated a certain number of times. Although, the FOR loop can do the same job but do the counting for you.
for i:=1 to 3 do
writeln(i);
This loop assigns the value 1 to the variable to begin with, and then counts up to 3 as it outputs each number turn by turn. The next example shows how the same can be applied to character variables (assigned as char), and also how loops can count down as well as up.
for mycharacter:='D' downto 'A' do
writeln(mycharacter);
This loop should result in the characters D, C, B and A to be output on screen in this exact order.
The last type of loop (REPEAT) executes code on at least one occasion, and then a check is made with an ending conditional statement. In contrast, the conditional statement is marked using the word UNTIL, and if the condition is true then the loop is stopped.
a:=1;
repeat
writeln(a);
a:=a+1;
until (a>3)
end;
Like the other examples, the variable a is output to the console with each iteration of the loop. The variable is incremented by one with each iteration until the number is larger than 3. Therefore 1, 2, and 3 are all written out on different lines.
In the next section of this tutorial you will learn how to organise your programs by sectioning parts of code into procedures or functions.
The Computer Monkey is a blog loosely based around the modern world, the Internet, computing and advancing, yet as ever new but possibly ludicrous technologies. Where an area strikes me with interest or inspiration, I'll write up about it to share with you readers and with a bit of luck you'll enjoy reading it!
Tuesday 3 November 2009
Saturday 31 October 2009
Delphi Tutorial Part 2
Conditional Statements
So far we have made a program to execute a bunch of fixed commands, so no matter what happens, all of the statements in that program will be carried out in chronological order. There are many cases though where we only want to carry out actions under certain cases or conditions, and this exactly what we're going to do in this part of the tutorial. The statements IF, ELSE and CASE will be demonstrated here to bring out a decision based element in our program. To do this, we will request an input about someones age and provide either a younger or an older user response.
Whenever we want to make a simple decision we use IF. The statements that follow IF are considered to be the conditions or expressions.
If (a=1) then
Begin
writeln('a equals 1');
end;
All of the commands after then are executed if the conditions turn out to be true. Above you can see that if the variable a equals 1, then the actions between begin and end are executed. In this scenario, the message "a equals 1" is output on screen. As this is an If statement though, and not the end of our program, END has to be terminated with a semi-colon.
You can choose to declare more than one condition in an IF statement, by using more than one group of brackets. We also have to separate two or more conditions using operators like OR, AND, XOR. The code below is an example of how we do this, and how much of a difference these operators can make.
Using the OR operator
If (a=1) OR (b=1) then
begin
writeln('either a or b equal 1');
end;
Using the AND operator
If (a=1) AND (b=1) then
begin
writeln('both a and b equal 1');
end;
In the first case, either a or b can be equal to one for the If statement to be true. Although with the second case, both a and b have to be equal to one for the if to be true. If the statement is in fact false, we can also choose to bind some instructions to this false condition using ELSE. ELSE in programming is just like saying, otherwise do this...
If (a=1) AND (b=1) then
begin
writeln('both a and b equal 1');
end
else
begin
writeln('either a or b does not equal 1');
end;
Now you can see that if a or b isn't equal to one, then a message is written on screen declaring that this is the case. However now that we have used the ELSE statement, we also have to shift the semi-colon from the IF clause down to the ELSE clause. Even better, you can choose to remove begin and end from both of these clauses if there is only one line inside them, like so.
If (a=1) AND (b=1) then
writeln('both a and b equal 1')
else
writeln('either a or b does not equal 1');
Here is a list of most of all the possible operators you can use in Delphi, with a small explanation of the purpose of each. Most of these are to be used in actual expressions like an equals sign, unlike the example above where AND/OR are used to join up expressions.
AND: both expressions on either side of this operator must be true to execute the code.
OR: one of the expressions on either side of the operator must be true to execute the code.
XOR: the expression on the left must be true, or the expression on the right must be false.
NOT: the expression that follows must be false for the condition to be true.
=: the left side of an expression or equation must be exactly equal to the right side for it to be true.
<: the left side of the equation must be less than the right side for the condition to be true.
<=: the left side of the equation must be less than or equal to the right side to be true.
>=: the left side of the equation must be larger or equal to the right side to be true.
=!: the left side of the equation must not be equal to the value on the right to be true.
<>: the left and right side of the equation cannot be equal to each other for the condition to be true.
You can go a step further with the IF and ELSE statements by rooting them off one another.
if (a=1) then
begin
if (b=1) then
writeln('both a and b equal 1')
else
writeln('just a equals 1');
end
else
if (b<>1) then
writeln('both a and b don't equal 1');
Sometimes you can have a ridiculous number of these statements, and so the CASE statement exists to simplify things greatly.
Case a of
1: writeln('a equals 1'); //case 1
2: writeln('a equals 2'); //case 2
3: writeln('a equals 3'); //case 3
else
writeln('a is less than 1 or larger than 3');
The only problem with the case statement, is that you can't use a string variable. You have to use an ordinal or fixed variant, such as an Integer.
Now that you have got to grips with how conditional statements work, hopefully you should understand the new code we will be adding below to our program.
program MyFirstProgram;
var
nameVar: String; //name variable
age: Integer;
begin
writeln('What is your name? ');
readln(nameVar);
writeln('Hello '+nameVar+' how old are you?');
//new code starts here
readln(age);
if age<23 then
writeln('Oh, so do you still study?')
else
writeln('What do you do for living?');
end.
We've added a new variable called age to use in our conditional statement, and have classed it as an integer as we only want to store a whole number. We then use IF to see if the age that was input, was less than 23 and then proceed accordingly. If the age was less than 23 we ask if this person is still studying, but otherwise we ask what they do for a living.
The next chapter in this tutorial covers loops, and how a process can be repeated as often as you want without re-typing code.
So far we have made a program to execute a bunch of fixed commands, so no matter what happens, all of the statements in that program will be carried out in chronological order. There are many cases though where we only want to carry out actions under certain cases or conditions, and this exactly what we're going to do in this part of the tutorial. The statements IF, ELSE and CASE will be demonstrated here to bring out a decision based element in our program. To do this, we will request an input about someones age and provide either a younger or an older user response.
Whenever we want to make a simple decision we use IF. The statements that follow IF are considered to be the conditions or expressions.
If (a=1) then
Begin
writeln('a equals 1');
end;
All of the commands after then are executed if the conditions turn out to be true. Above you can see that if the variable a equals 1, then the actions between begin and end are executed. In this scenario, the message "a equals 1" is output on screen. As this is an If statement though, and not the end of our program, END has to be terminated with a semi-colon.
You can choose to declare more than one condition in an IF statement, by using more than one group of brackets. We also have to separate two or more conditions using operators like OR, AND, XOR. The code below is an example of how we do this, and how much of a difference these operators can make.
Using the OR operator
If (a=1) OR (b=1) then
begin
writeln('either a or b equal 1');
end;
Using the AND operator
If (a=1) AND (b=1) then
begin
writeln('both a and b equal 1');
end;
In the first case, either a or b can be equal to one for the If statement to be true. Although with the second case, both a and b have to be equal to one for the if to be true. If the statement is in fact false, we can also choose to bind some instructions to this false condition using ELSE. ELSE in programming is just like saying, otherwise do this...
If (a=1) AND (b=1) then
begin
writeln('both a and b equal 1');
end
else
begin
writeln('either a or b does not equal 1');
end;
Now you can see that if a or b isn't equal to one, then a message is written on screen declaring that this is the case. However now that we have used the ELSE statement, we also have to shift the semi-colon from the IF clause down to the ELSE clause. Even better, you can choose to remove begin and end from both of these clauses if there is only one line inside them, like so.
If (a=1) AND (b=1) then
writeln('both a and b equal 1')
else
writeln('either a or b does not equal 1');
Here is a list of most of all the possible operators you can use in Delphi, with a small explanation of the purpose of each. Most of these are to be used in actual expressions like an equals sign, unlike the example above where AND/OR are used to join up expressions.
AND: both expressions on either side of this operator must be true to execute the code.
OR: one of the expressions on either side of the operator must be true to execute the code.
XOR: the expression on the left must be true, or the expression on the right must be false.
NOT: the expression that follows must be false for the condition to be true.
=: the left side of an expression or equation must be exactly equal to the right side for it to be true.
<: the left side of the equation must be less than the right side for the condition to be true.
<=: the left side of the equation must be less than or equal to the right side to be true.
>=: the left side of the equation must be larger or equal to the right side to be true.
=!: the left side of the equation must not be equal to the value on the right to be true.
<>: the left and right side of the equation cannot be equal to each other for the condition to be true.
You can go a step further with the IF and ELSE statements by rooting them off one another.
if (a=1) then
begin
if (b=1) then
writeln('both a and b equal 1')
else
writeln('just a equals 1');
end
else
if (b<>1) then
writeln('both a and b don't equal 1');
Sometimes you can have a ridiculous number of these statements, and so the CASE statement exists to simplify things greatly.
Case a of
1: writeln('a equals 1'); //case 1
2: writeln('a equals 2'); //case 2
3: writeln('a equals 3'); //case 3
else
writeln('a is less than 1 or larger than 3');
The only problem with the case statement, is that you can't use a string variable. You have to use an ordinal or fixed variant, such as an Integer.
Now that you have got to grips with how conditional statements work, hopefully you should understand the new code we will be adding below to our program.
program MyFirstProgram;
var
nameVar: String; //name variable
age: Integer;
begin
writeln('What is your name? ');
readln(nameVar);
writeln('Hello '+nameVar+' how old are you?');
//new code starts here
readln(age);
if age<23 then
writeln('Oh, so do you still study?')
else
writeln('What do you do for living?');
end.
We've added a new variable called age to use in our conditional statement, and have classed it as an integer as we only want to store a whole number. We then use IF to see if the age that was input, was less than 23 and then proceed accordingly. If the age was less than 23 we ask if this person is still studying, but otherwise we ask what they do for a living.
The next chapter in this tutorial covers loops, and how a process can be repeated as often as you want without re-typing code.
Thursday 29 October 2009
Delphi Tutorial Part 1
Variables
Variables in programming are used to hold useful bits of information. Numbers, characters, words, lists, memos, and any kind of data that needs to be referenced to, should have an associated variable. Variables in Computing also need to be assigned to different classes. These will be covered in a latter part of the tutorial, but in summary, they tell us what kind of data input there should be, and restrain us from using inappropriate data. In Delphi for example, the string class holds 225 bytes of data (255 characters), the integer class can hold any number between -2147483648 and 2147483647, and the double class can hold a decimal value up to 308 or negative 308 base 10. These three classes are the only ones you will need for now, but other very useful classes will covered in the course of this tutorial. A variable should proceed var in the main program with a unique name, followed by a colon and the class. Below I have wrote a sample program to show how a variable can be used to store your name, and give you a welcome message.
program MyFirstProgram;
var
nameVar: String; //name variable
begin
writeln('What is your name? ');
readln(nameVar);
writeln('Hello '+nameVar+' how are you doing today?');
readln();
end.
Since you probably only just discovered the language five minutes ago, we will need to cover some syntax here.
Every application in Pascal must begin with the word Program and there by followed by the name you would like to assign to it. This is our first command in our program, so therefore like any other instruction we need to terminate the command with a semi-colon. After this we declare our list of variables following var and then we initiate the main body of our program with the word begin. Thankfully we end our program just as easily using the word end. Programmers often refer to these as the beginning and the end of a clause. writeln() is used to output text to the screen, and an input is taken from the next line using readln() where hopefully the user should type in their name. Note, the name of the variable has been placed in between the brackets in order to return the value we need. You may notice at the end of of our writeln statement, we have added text to the end of it by using two '/' characters. This is known as a comment, and in bigger and more commercial situations these permit us to post reminders or pointers for you or any colleagues you may have. Then lastly, the writeln command is used again to output text but with the addition of the user's name. A variable here can almost be treated like a number, the name is inserted just by using addition signs and the name of the variable. The final line uses a read command with no output variable, to ensure that the text can be read by the person. Without this the program would just instantly end on the input of your name.
Variables of the Integer or floating point class can be treated similarly. The main difference is that only values can be added to them as opposed to text. We could have a variable name called a, and then assign a value to this using an equals sign. Likewise we could do the same with a variable named b, add the two together and then return this value to a third variable (called c maybe).
program AnotherProgram;
var
a: Integer;
b: Integer;
c: Integer;
begin
a:=1;
b:=1;
c:=a+b;
writeln(c);
readln();
end.
Delphi was made to assign values to variables by using the colon and the equals sign, but it is worth remembering that the standard is just the equals sign.
The next part of the tutorial will be about conditional statements, to help you understand how to trigger actions under certain events where necessary.
Variables in programming are used to hold useful bits of information. Numbers, characters, words, lists, memos, and any kind of data that needs to be referenced to, should have an associated variable. Variables in Computing also need to be assigned to different classes. These will be covered in a latter part of the tutorial, but in summary, they tell us what kind of data input there should be, and restrain us from using inappropriate data. In Delphi for example, the string class holds 225 bytes of data (255 characters), the integer class can hold any number between -2147483648 and 2147483647, and the double class can hold a decimal value up to 308 or negative 308 base 10. These three classes are the only ones you will need for now, but other very useful classes will covered in the course of this tutorial. A variable should proceed var in the main program with a unique name, followed by a colon and the class. Below I have wrote a sample program to show how a variable can be used to store your name, and give you a welcome message.
program MyFirstProgram;
var
nameVar: String; //name variable
begin
writeln('What is your name? ');
readln(nameVar);
writeln('Hello '+nameVar+' how are you doing today?');
readln();
end.
Since you probably only just discovered the language five minutes ago, we will need to cover some syntax here.
Every application in Pascal must begin with the word Program and there by followed by the name you would like to assign to it. This is our first command in our program, so therefore like any other instruction we need to terminate the command with a semi-colon. After this we declare our list of variables following var and then we initiate the main body of our program with the word begin. Thankfully we end our program just as easily using the word end. Programmers often refer to these as the beginning and the end of a clause. writeln() is used to output text to the screen, and an input is taken from the next line using readln() where hopefully the user should type in their name. Note, the name of the variable has been placed in between the brackets in order to return the value we need. You may notice at the end of of our writeln statement, we have added text to the end of it by using two '/' characters. This is known as a comment, and in bigger and more commercial situations these permit us to post reminders or pointers for you or any colleagues you may have. Then lastly, the writeln command is used again to output text but with the addition of the user's name. A variable here can almost be treated like a number, the name is inserted just by using addition signs and the name of the variable. The final line uses a read command with no output variable, to ensure that the text can be read by the person. Without this the program would just instantly end on the input of your name.
Variables of the Integer or floating point class can be treated similarly. The main difference is that only values can be added to them as opposed to text. We could have a variable name called a, and then assign a value to this using an equals sign. Likewise we could do the same with a variable named b, add the two together and then return this value to a third variable (called c maybe).
program AnotherProgram;
var
a: Integer;
b: Integer;
c: Integer;
begin
a:=1;
b:=1;
c:=a+b;
writeln(c);
readln();
end.
Delphi was made to assign values to variables by using the colon and the equals sign, but it is worth remembering that the standard is just the equals sign.
The next part of the tutorial will be about conditional statements, to help you understand how to trigger actions under certain events where necessary.
Wednesday 28 October 2009
A world without the Internet
Some have wondered what the world would come to if the Internet just suddenly disappeared. Some would become jobless; large online businesses like Google and Yahoo would collapse; people could lose contact, and generally the world would be a duller place. Over at www.cracked.com people have posted their own visions of a World tomorrow, without the Internet. People have been rewarded for their works in a photoshop competition.
The World tomorrow if the Internet disappeared
The World tomorrow if the Internet disappeared
Monday 26 October 2009
How to start Programming
There are many ways to which someone can choose to program, but I think a lot of people don't realise the potential that is out there. People can choose to do electronic programming which is known as low-level programming, or develop software of their own (high-level programming). Both of which are now widely available, but nevertheless the processes carried out in the two are different. Without a doubt, programming is easier and more flexible than people think or make it out to be. At the most, with a month's learning anyone can grasp the basics, start to build a business application or even start to build their own game. The beauty of programming is that you don't need encyclopedic knowledge of a Computer language to make a application or software solution, but the logic and the techniques you apply are the key. Programming is almost like a puzzle. Anyone can solve one over time, but what is the quickest way to do it? This blog focuses on this software orientated side of programming, and hopefully after reading this, you will feel more comfortable in choosing a language and starting to write some basic programs yourself.
What makes a good programmer?
A good programmer is usually distinguished by his or her ability in logical thinking. Despite what some people think, Maths is not largely involved until someone decides to delve into fields like Artificial Intelligence, or 3D programming. Even in these cases, the methods applied relate to formulae that have already been found out and provided for us. So, don't let this put you off. However, if you seriously want to make programming a career, patience is essential. There will at some point come a time, where theres a problem with your work, or you are simpy unsure how to implement. Problems like these that arise like this can be very frustrating at times, and can take many hours to solve. It will take persistence and some visualisation to see through these situations and debug your programs. Those who can visualise and seperate their programs into neat, logical and simple instructions are one step ahead into thinking like programmers.
What Computer Language should I write in?
There are so many languages to choose from now, it can be quite hard to conclude which language is best for you and your programs. Pascal/Delphi, Python and Visual Basic are what I believe to be some of the best beginner programming languages out there. Both Borland Pascal/Delphi and Visual basic come with good interfaces, and are packaged with the .NET framework for you to instantly make your own Windows applications. All of which are easy to get to know, Python in particular, and all of them having thriving online communities with the exception of Delphi. Pascal/Delphi has roots that go back as far as the 1980's, hence why there is a lack of online support. Although, I still think it is a logical language to start learning with, even if some dislike the syntax and structure it uses. I intend to bring back some of its delights with a small 10 step guide below into learning Delphi.
10 steps to understanding Delphi
I have decided to release a quick Delphi tutorial in different posts, over the course of a few days. Before you begin this guide though you will need to download and install a compiler. Unfortunately, in the process of writing this post I found out that Borland Delphi 2006 is no longer available for free download. Two good alternatives though can be found here and here.
Two very good sources on Delphi are Delphi.about.com and Delphibasics.co.uk if you choose to gather more in depth knowledge. I will also be posting some of my own projects in the future for people to try out for themselves.
What makes a good programmer?
A good programmer is usually distinguished by his or her ability in logical thinking. Despite what some people think, Maths is not largely involved until someone decides to delve into fields like Artificial Intelligence, or 3D programming. Even in these cases, the methods applied relate to formulae that have already been found out and provided for us. So, don't let this put you off. However, if you seriously want to make programming a career, patience is essential. There will at some point come a time, where theres a problem with your work, or you are simpy unsure how to implement. Problems like these that arise like this can be very frustrating at times, and can take many hours to solve. It will take persistence and some visualisation to see through these situations and debug your programs. Those who can visualise and seperate their programs into neat, logical and simple instructions are one step ahead into thinking like programmers.
What Computer Language should I write in?
There are so many languages to choose from now, it can be quite hard to conclude which language is best for you and your programs. Pascal/Delphi, Python and Visual Basic are what I believe to be some of the best beginner programming languages out there. Both Borland Pascal/Delphi and Visual basic come with good interfaces, and are packaged with the .NET framework for you to instantly make your own Windows applications. All of which are easy to get to know, Python in particular, and all of them having thriving online communities with the exception of Delphi. Pascal/Delphi has roots that go back as far as the 1980's, hence why there is a lack of online support. Although, I still think it is a logical language to start learning with, even if some dislike the syntax and structure it uses. I intend to bring back some of its delights with a small 10 step guide below into learning Delphi.
10 steps to understanding Delphi
I have decided to release a quick Delphi tutorial in different posts, over the course of a few days. Before you begin this guide though you will need to download and install a compiler. Unfortunately, in the process of writing this post I found out that Borland Delphi 2006 is no longer available for free download. Two good alternatives though can be found here and here.
Two very good sources on Delphi are Delphi.about.com and Delphibasics.co.uk if you choose to gather more in depth knowledge. I will also be posting some of my own projects in the future for people to try out for themselves.
Sunday 25 October 2009
Will Windows 7 be a hit? Will it work?
A few years ago, many of us faced a huge disappointment from Microsoft's Operating System Windows Vista. Like the rest of their Operating Systems it was widely anticipating a release, but soon after, it was also widely regarded as one of Microsoft's biggest let downs. Vista brought to us a completely new and more attractive interface, but it also brought bugs, security issues, high hardware requirements and of course dreadfully slow file transfers. On the other hand however, maybe Microsoft has had a bit too much abuse recently. Vista was a completely new system after all, so their team of developers all had to start from the ground up. Taking this into account, it is no wonder why fixes and adjustments had to be made, and Microsoft have been very busy, and very quick at releasing Windows 7 to rectify this. With the release of Windows 7 last Friday I am sure that many of us are keen to know if it does exactly this. Critics have provided some promising reviews, and I know some who still wished they were using the beta over Windows Vista. The review from CNET.com and endadget.com provide some detailed reviews for those who are interested to find out.
Friday 23 October 2009
Add a Dynamic domain name to your website

In response to the article How to turn an old PC into a website the dilemma which many home setups face, is the fact that most people will have dynamic IP address. This means, whenever you choose to disconnect and connect to your Internet Service Provider you will effectively be given a different address. The domain name or the web address which it is attached to becomes invalid, and typing that address into your web browser, will just provide you with an error message. It's almost like knocking on someones door to later find out they have moved away, but in this case they have moved without choice. When a site is attached to such an address, this can be problematic, and holding a connection by say leaving your router on for the purpose of your site is very impractical.
To counter this problem you could always ask your ISP to give you a static/fixed IP address but this normally comes with a premium. However, this is where dynamic domain names come into play. A service such as DynDNS can provide you with a free domain, or a more desirable one if you choose to pay for it, and then keep track of your website location by using their software.
First of all you will need to sign up to their service in order to use one of their permitted domains. There after, all you need to do is login, go to "My Account" and then select "Add Host Service". Enter the domain name of choice, and add the entry. Download the Updater from here, install it and all that should remain is to enter your account and domain details. DynDNS should do the rest of the work for you.
Thursday 22 October 2009
How to turn an old PC into a website
There comes a point in life where your PC is just not up to the job anymore. Chances are this Computer is just sitting there in the garage, collecting dust as time passes, just waiting for you to chuck it in the tip. However, instead of it just lying there, why not turn it into something useful? When it comes to Computers, no matter how slow it may seem today, it was built for a purpose. One particular task which pretty much any Computer can do, is act as a Web Server. If you plan on making a relatively small or medium site, then neither processing or Bandwidth should be a problem. Without content such as videos and images, a typical web page is only about 60kb after all. This article will take you through some small, and simple steps to get your server up and running so you can host your own Website.
1. Firstly, you will need the right software in order to run your server. The best option available is Apache, but often this can seem a bit confusing for beginners and it isn't always configured to people's needs. A package known as XAMPP is a very simple and neat program which can hide any of these complications, and it can be downloaded from here.
2. If you haven't already, add a firewall rule for the XAMPP program you are using and open tcp ports 80 and 3306. You should access the Control Panel and start the Apache and MYSQL modules. Note if you want these to start up these automatically when your Computer starts, you will need to add these as Services via the Services button on the panel. SQL is also not required, but you will need it if you intend to store data, add a user login, or a forum to your site.
3. In your web browser, type in "http://localhost/security/xamppsecurity.php" and change the password for the MYSQL database, and also add a username and password for the XAMPP directory. This will make sure no-one on your network, or anywhere on the Internet can access the pages you are seeing, or edit any important files.
4. Next you will need to ensure that the correct ports are open on your router so that people can see your site across the Internet. For most routers, type into your browser "http://192.168.0.1", or "http://192.168.1.0" if you are using a Belkin router like the one in this tutorial. You should look for a link flagged as "Virtual Servers", "ports", "security", or just simply "firewall". Add an inbound firewall rule for ports 80 and 3306, and point it to your Server's IP address. If you don't know your local ip address, type in "ipconfig" into the command prompt and it should be labelled as the "IPv4 address".
5. Finally, you need to go to the XAMPP directory on your computer (often located at C:/Program Files/XAMPP), go to htdocs and change the index page to your site homepage. It is important that your homepage is named index in order for your site to be found directly. Any images, or files which you add to your site will need to be added here in the htdocs folder, and not outside of it or in the XAMPP sub-directory.
6. Now you have a website! Without content, and a domain name of course but nevertheless that's the bulk of the setup done and dusted. Just go to http://whatismyipaddress.com to find out what your global IP address is, and then type the same number into your browser. If you followed the instructions correctly, you should be able to see your web page. The very last step is adding a domain name to your site. I will be posting another article on how to create your own free domain name, and to make sure it keeps track of your own Server. Have Fun!
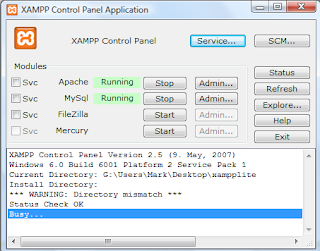
2. If you haven't already, add a firewall rule for the XAMPP program you are using and open tcp ports 80 and 3306. You should access the Control Panel and start the Apache and MYSQL modules. Note if you want these to start up these automatically when your Computer starts, you will need to add these as Services via the Services button on the panel. SQL is also not required, but you will need it if you intend to store data, add a user login, or a forum to your site.
3. In your web browser, type in "http://localhost/security/xamppsecurity.php" and change the password for the MYSQL database, and also add a username and password for the XAMPP directory. This will make sure no-one on your network, or anywhere on the Internet can access the pages you are seeing, or edit any important files.
4. Next you will need to ensure that the correct ports are open on your router so that people can see your site across the Internet. For most routers, type into your browser "http://192.168.0.1", or "http://192.168.1.0" if you are using a Belkin router like the one in this tutorial. You should look for a link flagged as "Virtual Servers", "ports", "security", or just simply "firewall". Add an inbound firewall rule for ports 80 and 3306, and point it to your Server's IP address. If you don't know your local ip address, type in "ipconfig" into the command prompt and it should be labelled as the "IPv4 address".
5. Finally, you need to go to the XAMPP directory on your computer (often located at C:/Program Files/XAMPP), go to htdocs and change the index page to your site homepage. It is important that your homepage is named index in order for your site to be found directly. Any images, or files which you add to your site will need to be added here in the htdocs folder, and not outside of it or in the XAMPP sub-directory.
6. Now you have a website! Without content, and a domain name of course but nevertheless that's the bulk of the setup done and dusted. Just go to http://whatismyipaddress.com to find out what your global IP address is, and then type the same number into your browser. If you followed the instructions correctly, you should be able to see your web page. The very last step is adding a domain name to your site. I will be posting another article on how to create your own free domain name, and to make sure it keeps track of your own Server. Have Fun!
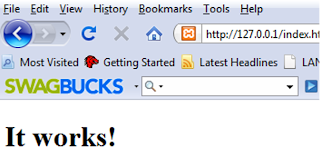
Wednesday 21 October 2009
Water doesn't spell the end for for Computers!
There is nothing worse than making yourself a nice cup of coffee, before waving your hand, and then spilling it all over your desk. It is just as frustrating however, if not more frustrating, to spill it all over a device of yours, a computer keyboard or even an entire Laptop. Cases like these are hardly rare, but inevitably, most of us won't pay any attention to them until it actually happens... or something breaks. This is why I decided to write some suggestions.
In case you didn't know, spilling water over any electronics won't affect it unless the power is on. The reason why liquids make devices go wrong in the first place, is because residue forms and the electronics are short circuited. So, in a last ditch attempt to save your gadget, laptop, keyboard or whatever it maybe, turn it upside down and turn it off.
Surprisingly enough, it can take days or even a week depending on the severity of the situation to get all of the water out. You should keep the device, upside down and turned off at all times in a humid environment. Do not place it in front of anything that directly radiates heat, such as a blow dryer or a radiator. This could cause further damage to the product if you decided to do this. Last but not least, as wrong as it may seem, use water! If it was one of those sticky drinks that you spilled, it is almost vital to use water to cleanse the device before you start drying it. This could be very difficult for those of you with gadgets, sorry guys. If it is a keyboard or a laptop you are drying, I would also suggest to take out some of the keys.
In case you didn't know, spilling water over any electronics won't affect it unless the power is on. The reason why liquids make devices go wrong in the first place, is because residue forms and the electronics are short circuited. So, in a last ditch attempt to save your gadget, laptop, keyboard or whatever it maybe, turn it upside down and turn it off.
Surprisingly enough, it can take days or even a week depending on the severity of the situation to get all of the water out. You should keep the device, upside down and turned off at all times in a humid environment. Do not place it in front of anything that directly radiates heat, such as a blow dryer or a radiator. This could cause further damage to the product if you decided to do this. Last but not least, as wrong as it may seem, use water! If it was one of those sticky drinks that you spilled, it is almost vital to use water to cleanse the device before you start drying it. This could be very difficult for those of you with gadgets, sorry guys. If it is a keyboard or a laptop you are drying, I would also suggest to take out some of the keys.
Tuesday 20 October 2009
Text speak or Geek speak?
Everyone knows what I'm talking about it. Those funny but sometimes ambigous abbreviations for phrases. I am certainlly guilty as charged when it comes to using them, and I'm sure you are too! They are so handy for short and quick mssages, that we all have adapted to using them over the years of sitting in front of a keyboard, or texting away with friends. The trouble is, the more you use them, you more likely you start to use them in odd places. I just used one then, "texting", but shouldn't that be a new verb anyway? I remember at times at secondary school, where I used to write "r" and "u" in my English essays without even realising it! Is this just one of those youthful mistakes? I for one am not sure. It hasn't cropped up again since then, but it has appeared in speech. Even today, I have mentioned such terms like "omg" in real life speech and it's not uncommon to recall others doing exactly the same. So who knows, maybe these words could be part of the dictionary one day, but in the mean time, they are stuck in the reserve of technology, so why not "lol" to this.
Monday 19 October 2009
Does Online Dating work?
The World is an ever shrinking one, courtesy of our old good friend called the Internet. So how can it get any smaller? The answer not only lies in social networking sites such as Facebook and Myspace, but online dating. From the depths of a community full of black hat hackers, malicious spyware and enough viruses to keep the entire world behind closed doors, can we really trust anyone on the Internet? The prospect of a distant relationship in the past let alone an online relationship, has neither been the most traditional, or one of the most intimate dating possibilities available to an individual. It is for these very reasons that people can choose to point the finger and laugh. The reality is a concept which has headed in the wrong direction, and misguided by these people and fake partners half way across the world.
The word "Love" itself is an abstract noun. In other words, something that cannot be physically sensed by a human being. This idea I think is completely symbolic of online dating. If love is nothing but a human concept, how cannot anyone be in love? After all, Love is without boundaries right? Some people may find an online relationship humorous, but these people are the ones missing out on an opportunity. The beauty of online dating, is how much selection you have. You literally have a selection of thousands, and a bunch of renown sites to choose from. Life is never ideal, and Love doesn't always come in neat packages, like a close friend or an instance of Love at first sight. If you want to find a perfect match, you are just as likely, if not more likely to find it on the Web contrary to belief.
I myself have voyaged into online dating during this last week, and I can say it just takes some time to find the right person, and even the right website. I hope others won't feel restrained to do the same (if you are single of course) and see for yourself how well it does work out for you. It is not for everyone, and at times it can be a gamble. Persistence does pay dividends though. Spend a good deal of time getting to know people, ensure you choose a more serious site, and you should be able to distinguish between a cardboard cutout and a possible partner.
I suggest to follow this link and this link, to find a list of reliable dating sites for you to try out. Here is hoping that you all find the date of your dreams!
The word "Love" itself is an abstract noun. In other words, something that cannot be physically sensed by a human being. This idea I think is completely symbolic of online dating. If love is nothing but a human concept, how cannot anyone be in love? After all, Love is without boundaries right? Some people may find an online relationship humorous, but these people are the ones missing out on an opportunity. The beauty of online dating, is how much selection you have. You literally have a selection of thousands, and a bunch of renown sites to choose from. Life is never ideal, and Love doesn't always come in neat packages, like a close friend or an instance of Love at first sight. If you want to find a perfect match, you are just as likely, if not more likely to find it on the Web contrary to belief.
I myself have voyaged into online dating during this last week, and I can say it just takes some time to find the right person, and even the right website. I hope others won't feel restrained to do the same (if you are single of course) and see for yourself how well it does work out for you. It is not for everyone, and at times it can be a gamble. Persistence does pay dividends though. Spend a good deal of time getting to know people, ensure you choose a more serious site, and you should be able to distinguish between a cardboard cutout and a possible partner.
I suggest to follow this link and this link, to find a list of reliable dating sites for you to try out. Here is hoping that you all find the date of your dreams!
Subscribe to:
Posts (Atom)